This is a simple gear wheels example, each gear is created by two rectangles in a specific degree and spinning around. A RotateTransition
is used to rotate both rectangles by 360 degrees repeatedly over a period of 5000 milliseconds, creating the illusion of both rectangles spinning together. All gears will be rotating in the middle of the window and keep moving in the same speed. The blue gear has a negative direction.
Maven pom.xml with JavaFX dependencies:
<dependencies> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-controls</artifactId> <version>17.0.2</version> </dependency> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-fxml</artifactId> <version>17.0.2</version> </dependency> <!-- other dependencies --> </dependencies>
Java code:
package com.example.testjavafx; import javafx.animation.Animation; import javafx.animation.RotateTransition; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Rectangle; import javafx.stage.Stage; import javafx.util.Duration; public class GearWheels extends Application { private static final int WIDTH = 800; private static final int HEIGHT = 600; private static final int SIZE = 100; private static final int TEETH = 10; private static final int ROTATION_TIME = 5000; @Override public void start(Stage primaryStage) { Group root = new Group(); createGear(95, 95, Color.RED,45, 0, 360, root); createGear(185, 185, Color.BLUE, 60, 15, -360, root); createGear(270, 95, Color.ORANGE, 45, 0, 360, root); Scene scene = new Scene(root, WIDTH, HEIGHT); primaryStage.setScene(scene); primaryStage.show(); } private static void createGear(int x, int y, Color color, double r1, double r2, int direction, Group root) { Rectangle rect5 = new Rectangle(x, y, SIZE, SIZE); rect5.setArcHeight(SIZE / TEETH); rect5.setArcWidth(SIZE / TEETH); rect5.setFill(color); rect5.setRotate(r1); root.getChildren().addAll(rect5); Rectangle rect6 = new Rectangle(x, y, SIZE, SIZE); rect6.setArcHeight(SIZE / TEETH); rect6.setArcWidth(SIZE / TEETH); rect6.setFill(color); rect6.setRotate(r2); root.getChildren().addAll(rect6); gearMove(rect5, rect6, direction); } private static void gearMove(Rectangle rect1, Rectangle rect2, int direction) { RotateTransition rt1 = new RotateTransition(Duration.millis(ROTATION_TIME), rect1); rt1.setByAngle(direction); rt1.setCycleCount(Animation.INDEFINITE); rt1.play(); RotateTransition rt2 = new RotateTransition(Duration.millis(ROTATION_TIME), rect2); rt2.setByAngle(direction); rt2.setCycleCount(Animation.INDEFINITE); rt2.play(); } public static void main(String[] args) { launch(args); } }
Result:
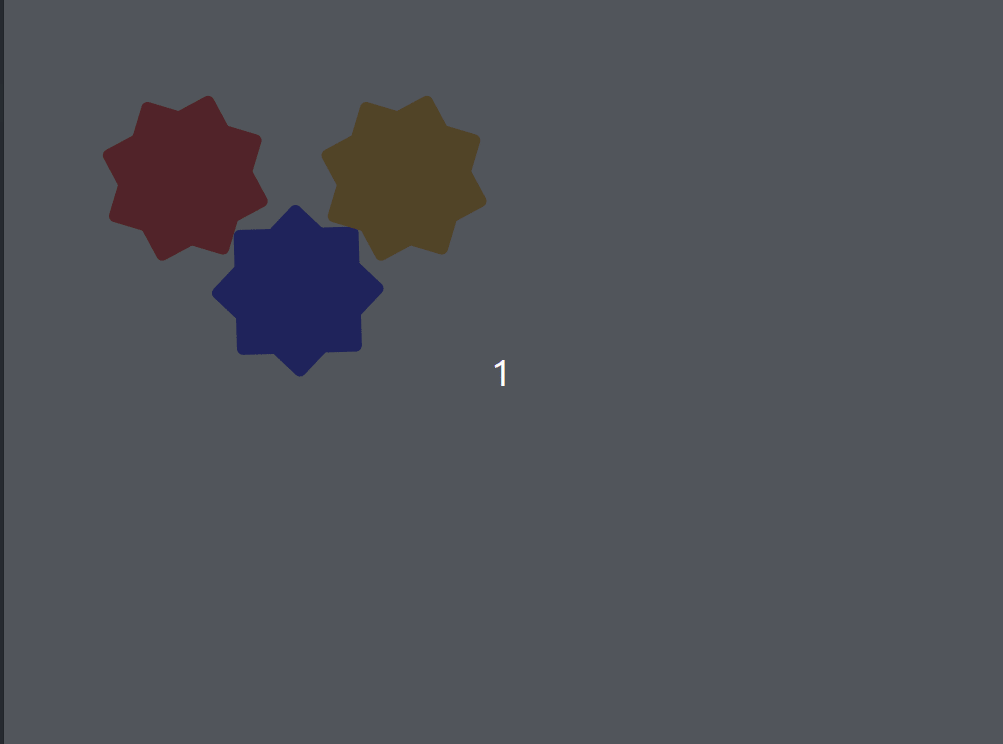