Simple Java FX application which creates and removes elements from the list. This is a basic example of a To Do List application using JavaFX. The program creates a window with a text field for inputting new tasks, a list view for displaying the tasks, and two buttons for adding and removing tasks. The tasks are stored in an observable list, which allows the list view to automatically update when tasks are added or removed. The tasks are only in memory and they do not in the hard disk.
Maven pom.xml file with JavaFX dependencies:
<dependencies> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-controls</artifactId> <version>17.0.2</version> </dependency> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-fxml</artifactId> <version>17.0.2</version> </dependency> <!-- other dependencies --> </dependencies>
Java code:
package com.example.testjavafx; import javafx.application.Application; import javafx.collections.FXCollections; import javafx.collections.ObservableList; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.ListView; import javafx.scene.control.TextField; import javafx.scene.layout.HBox; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class TodoList extends Application { private TextField taskInput; private ListView<String> taskList; private ObservableList<String> tasks; public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { primaryStage.setTitle("Todo List"); taskInput = new TextField(); taskInput.setPromptText("Enter a new task"); Button addButton = new Button("Add"); addButton.setOnAction(e -> addTask()); // make Add button default. // This way if we press Enter then it will be activated addButton.setDefaultButton(true); Button removeButton = new Button("Remove"); removeButton.setOnAction(e -> removeTask()); tasks = FXCollections.observableArrayList(); taskList = new ListView<>(tasks); HBox buttonLayout = new HBox(); buttonLayout.setSpacing(10); buttonLayout.getChildren().addAll(taskInput, addButton, removeButton); VBox layout = new VBox(); layout.setSpacing(10); layout.setPadding(new Insets(10, 10, 10, 10)); layout.getChildren().addAll(buttonLayout, taskList); Scene scene = new Scene(layout, 400, 300); primaryStage.setScene(scene); primaryStage.show(); } private void addTask() { String task = taskInput.getText(); if (!task.isEmpty()) { tasks.add(task); taskInput.clear(); } } private void removeTask() { int selectedIndex = taskList.getSelectionModel().getSelectedIndex(); if (selectedIndex != -1) { taskList.getItems().remove(selectedIndex); } } }
The result is:
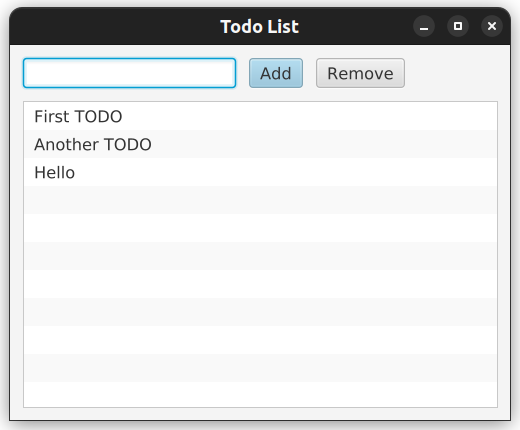