In this example we will use Java FX and create animation in which Earth will go around the sun:
Maven pom.xml dependencies of JavaFX:
<dependencies> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-controls</artifactId> <version>17.0.2</version> </dependency> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-fxml</artifactId> <version>17.0.2</version> </dependency> <!-- other dependencies --> </dependencies>
Java code:
package com.example.demo; import javafx.animation.Interpolator; import javafx.animation.PathTransition; import javafx.application.Application; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.scene.shape.Ellipse; import javafx.stage.Stage; import javafx.util.Duration; public class MainClass extends Application { @Override public void start(Stage primaryStage) { Group root = new Group(); // Create the sun Circle sun = new Circle(250, 150, 50, Color.YELLOW); // Create the Earth orbit Ellipse earthOrbit = new Ellipse(250, 150, 200, 100); earthOrbit.setStroke(Color.WHITE); earthOrbit.setFill(null); // Create the Earth Circle earth = new Circle(10, Color.BLUE); // Add the sun, Earth orbit, and Earth to the root node root.getChildren().addAll(sun, earthOrbit, earth); // Create the animation for the Earth PathTransition animation = new PathTransition(); animation.setDuration(Duration.seconds(10)); animation.setNode(earth); animation.setPath(earthOrbit); animation.setCycleCount(PathTransition.INDEFINITE); // The Interpolator.LINEAR will make the transition of the animation // linear instead of accelerating and slowing down. animation.setInterpolator(Interpolator.LINEAR); animation.play(); // Create the scene and show the stage Scene scene = new Scene(root, 500, 300, Color.BLACK); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
This program creates a simple animation of the Earth going around the Sun using the JavaFX library. The animation is created using a PathTransition object, which animates a node along a specified path. In this case, the path is an Ellipse object that represents the Earth’s orbit around the Sun. The Circle object represents the Earth and the Sun.
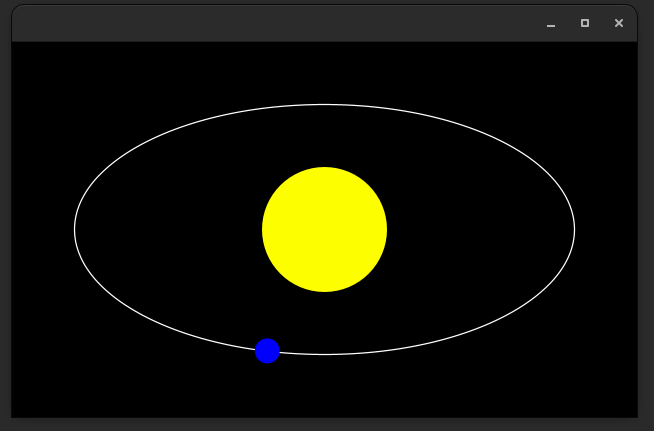