This is a simple example of a mp3 player created with JavaFX. It has a button to open and play the track, pause, resume and stop buttons with their respective functionalities. To play the music we use MediaPlayer class -> The MediaPlayer
class provides the controls for playing media.
MediaPlayer
provides the pause()
, play()
, stop()
and seek()
controls as well as the rate
and autoPlay
properties which apply to all types of media. It also provides the balance
, mute
, and volume
properties which control audio playback characteristics.
Maven pom.xml file with the JavaFX dependencies:
<dependencies> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-controls</artifactId> <version>17.0.2</version> </dependency> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-fxml</artifactId> <version>17.0.2</version> </dependency> <dependency> <groupId>org.openjfx</groupId> <artifactId>javafx-media</artifactId> <version>17.0.2</version> </dependency> <!-- other dependecies --> </dependencies>
Java code:
package com.example.testjavafx; import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.BorderPane; import javafx.scene.layout.HBox; import javafx.stage.FileChooser; import javafx.stage.Stage; import javafx.scene.media.Media; import javafx.scene.media.MediaPlayer; import java.io.File; public class MP3Player extends Application { MediaPlayer mediaPlayer; Label label = new Label("No file selected"); public static void main(String[] args) { launch(args); } @Override public void start(Stage stage) { Button openButton = new Button("Open"); openButton.setOnAction(event -> { FileChooser fileChooser = new FileChooser(); File file = fileChooser.showOpenDialog(stage); if (file != null) { String filePath = file.getAbsolutePath(); label.setText(filePath); Media media = new Media(new File(filePath).toURI().toString()); mediaPlayer = new MediaPlayer(media); mediaPlayer.play(); } }); Button pauseButton = new Button("Pause"); pauseButton.setOnAction(event -> { mediaPlayer.pause(); }); Button resumeButton = new Button("Resume"); resumeButton.setOnAction(event -> { mediaPlayer.play(); }); Button stopButton = new Button("Stop"); stopButton.setOnAction(event -> { mediaPlayer.stop(); }); BorderPane borderPane = new BorderPane(); HBox buttons = new HBox(openButton, pauseButton, resumeButton, stopButton); borderPane.setTop(buttons); borderPane.setCenter(label); BorderPane.setAlignment(label, Pos.CENTER); Scene scene = new Scene(borderPane, 650, 200); stage.setScene(scene); stage.setTitle("MP3 Player"); stage.show(); } }
Result:
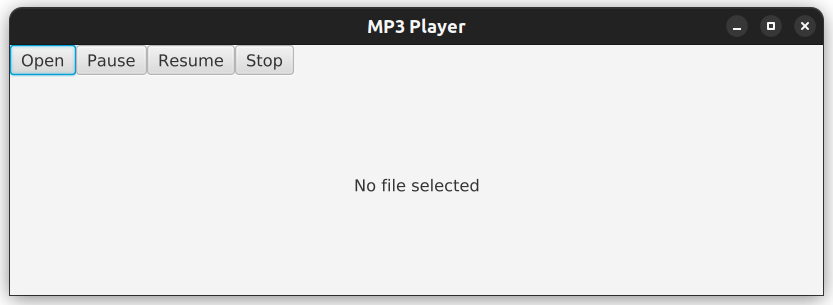