In this tutorial we can see how to create digital clock with Java FX.
Here is the project structure:
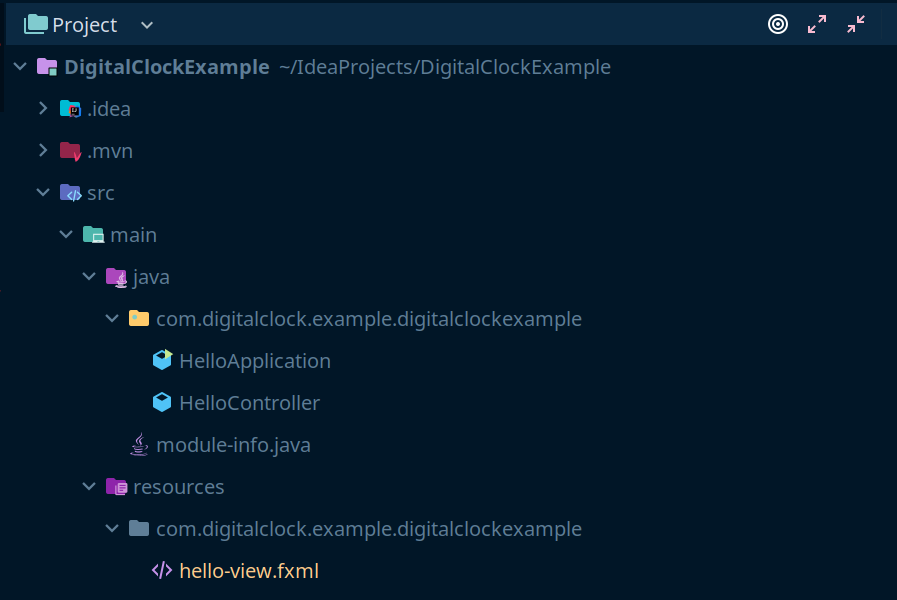
First let’s create see the HelloApplication which has the main method:
package com.digitalclock.example.digitalclockexample; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Scene; import javafx.stage.Stage; import java.io.IOException; public class HelloApplication extends Application { @Override public void start(Stage stage) throws IOException { FXMLLoader fxmlLoader = new FXMLLoader(HelloApplication.class.getResource("hello-view.fxml")); Scene scene = new Scene(fxmlLoader.load(), 220, 140); stage.setTitle("Digital clock"); stage.setScene(scene); stage.show(); } public static void main(String[] args) { launch(); } }
As you can see we extend Application and then provide implementation of start method. We use FXMLLoader to load hello-view.fxml which contains xml representation of the layout ant the components. The we create Scene and add it to the stage which is available in Application parent class.
Here is the hello-view.fxml:
<?xml version="1.0" encoding="UTF-8"?> <?import javafx.geometry.Insets?> <?import javafx.scene.control.Label?> <?import javafx.scene.layout.VBox?> <VBox fx:id="container" alignment="CENTER" spacing="20.0" xmlns:fx="http://javafx.com/fxml" fx:controller="com.digitalclock.example.digitalclockexample.HelloController"> <padding> <Insets bottom="20.0" left="20.0" right="20.0" top="20.0"/> </padding> <Label fx:id="clockLabel"/> </VBox>
We also need to create controller class which load and initialize the components:
package com.digitalclock.example.digitalclockexample; import javafx.animation.AnimationTimer; import javafx.fxml.FXML; import javafx.fxml.Initializable; import javafx.scene.control.Label; import javafx.scene.layout.VBox; import javafx.scene.text.Font; import java.net.URL; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.util.ResourceBundle; public class HelloController implements Initializable { public VBox container; @FXML private Label clockLabel; @Override public void initialize(URL url, ResourceBundle resourceBundle) { container.setStyle("-fx-background-color: black"); clockLabel.setStyle("-fx-text-fill: green"); clockLabel.setFont(Font.font("Classic Console", 50d)); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("hh:mm:ss"); AnimationTimer timer = new AnimationTimer() { @Override public void handle(long now) { LocalDateTime localDateTime = LocalDateTime.now(); clockLabel.setText(formatter.format(localDateTime)); } }; timer.start(); } }
In this case implement Initializable method initialize which will be called on time when the application is started. In side this method we set up the color of the background and the label text color and font size. We also create AnimationTimer instance implement method handle which will be called on every frame. Inside of this method we create LocalDateTime instance and we set it on the clockLabel which is the visible Java FX label. At the end we start the timer with timer.start().
The whole example you can find it in github